Unlock the power of Chrome extensions and elevate your web capture experience! In this step-by-step tutorial, we’ll guide you through the process of building a robust Chrome extension that effortlessly captures full-page screenshots, providing users with a seamless and enhanced browsing experience.
The tutorial will cover creating the necessary files, implementing the content script and popup script, and explaining the key components of the code.
Note : Remember that capturing full-page screenshots of all types of websites can be complex due to variations in web page structures and behaviors. This is a simplified example and might need to be adjusted based on your specific needs
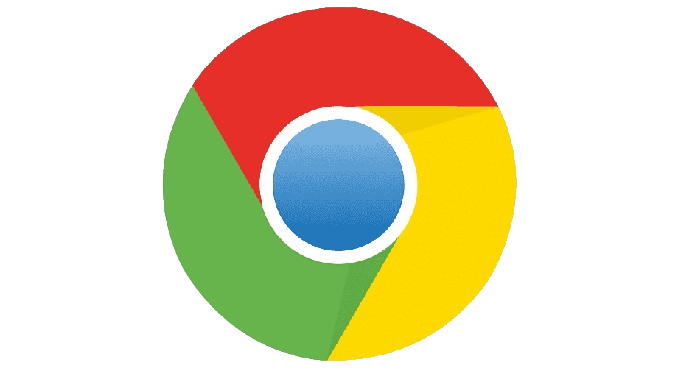
Step 1: Set Up Extension Files
- Create a new folder for your extension.
- Inside the folder, create the following files:
manifest.json
contentScript.js
popup.html
popup.js
background.js
Step 2: Configure manifest.json
Step 3: Create popup.html
Step 4: Implement content.js
Step 5: Implement popup.js
Step 6: Implement background.js
The source code for this project is available on Github.
High-Level Algorithm:
- Content Script (
contentScript.js
):- Listens for a message from the extension popup to initiate screenshot capture.
- Retrieves the dimensions of the webpage and the device pixel ratio.
- Initializes variables for tracking the captured height and storing captured images.
- Defines a function (
captureAndScroll
) to capture the visible area, scroll to the next part of the page, and repeat until the entire page is captured. - Sends a message to the background script to capture the visible tab and receives the captured image data.
- Checks for duplicate images to avoid capturing the same content multiple times.
- Scrolls to the next part of the page and continues the process until the entire page is captured.
- Sends back the complete array of captured image data to the popup.
- Popup Script (
popup.js
):- Listens for a click on the “Capture Screenshot” button in the popup.
- Queries the active tab to get its ID.
- Sends a message to the content script to initiate the screenshot capture.
- Receives the captured image data from the content script.
- Creates a canvas to stitch together the captured images.
- Sets the canvas width based on the width of the first captured image and the height based on the total height of all captured images.
- Draws each image onto the canvas, adjusting the Y-coordinate based on the index.
- Creates a download link for the full-page screenshot, triggers a click event, and removes the link from the document.
- Background Script (
background.js
):- Listens for a message from the content script with the action “captureVisibleTab.”
- Uses the
chrome.tabs.captureVisibleTab
API to capture the visible area of the tab in PNG format. - Sends the captured image data back to the content script.