In today’s fast-paced digital world, capturing and sharing moments from your web browser has become an essential part of our online experience. Whether it’s for creating tutorials, archiving web content, or simply sharing your online adventures with friends, having a reliable and user-friendly tool for recording your web browsing sessions can be a game-changer.
That’s where our Chrome extension, “Chrome Extension Manifest V3 Recording the Current Tab,” comes into play. This lightweight and intuitive extension, built using the latest Chrome Manifest V3 architecture, empowers you to effortlessly record the current tab in your Chrome browser. With just a few clicks, you can capture video footage of whatever you’re viewing on the web, making it an invaluable addition to your web toolkit.
In this blog post, we’ll dive into the features and functionalities of our Chrome extension, explore the motivation behind its creation, and guide you on how to get started with recording your web adventures. Whether you’re a content creator, educator, or simply someone who loves to document their online experiences, read on to discover how our extension can enhance your web browsing journey. Let’s embark on this exciting journey of capturing the web, one tab at a time.
You can checkout the demo of the extension
Here’s an example of how you can accomplish this:
- Update your manifest.json file:
{
"manifest_version": 3,
"name": "Tab Recorder",
"version": "1.0",
"description": "Record and display current tab",
"permissions": [
"activeTab",
"tabCapture"
],
"background": {
"service_worker": "background.js"
},
"action": {
"default_popup": "popup.html"
},
"content_scripts": [
{
"matches": ["<all_urls>"],
"js": ["content.js"]
}
]
}
- Create a popup.html file with the following content:
<!DOCTYPE html>
<html>
<head>
<style>
/* Inline CSS for styling */
body {
width: 210px;
padding: 20px;
text-align: center;
}
h1 {
font-size: 20px;
}
button {
background-color: #0073e6;
color: #fff;
border: none;
padding: 10px 20px;
margin: 10px;
cursor: pointer;
}
button:hover {
background-color: #005ba4;
}
</style>
</head>
<body>
<!-- Heading -->
<h1>Record Screen</h1>
<!-- Start Button -->
<button id="record">Start</button>
<!-- Stop Button -->
<button id="stop">Stop</button>
</body>
<script src="popup.js"></script>
</html>
- Create a popup.js file with the following content:
const onButtonClick = (event) => {
const button = event.target;
if (button.id === "record") {
window.chrome.tabs.query({ currentWindow: !0, active: !0 }, function (e) {
var n = e[0];
chrome.tabCapture.getMediaStreamId({consumerTabId: n.id}, (streamId) => {
chrome.tabs.sendMessage(n.id, {type:"tabRecord",streamId:streamId,tabId:n.id});
});
});
} else if (button.id === "stop") {
window.chrome.tabs.query({ currentWindow: !0, active: !0 }, function (e) {
var n = e[0];
window.chrome.tabs.sendMessage(n.id, { type: "stopRecording" });
});
}
};
document.getElementById("record").addEventListener("click", onButtonClick);
document.getElementById("stop").addEventListener("click", onButtonClick);
- Lastly Create a content.js file with the following content:
var mediaRecorder ;
var recordedChunks = [];
var seconds = 0
function stopRecord(recordedChunks){
if(mediaRecorder.state == 'recording'){
mediaRecorder.stop();
}
var blob = new Blob(recordedChunks, {
'type': 'video/mp4'
});
var url = URL.createObjectURL(blob);
const downloadLink = document.createElement('a');
// Set the anchor's attributes
downloadLink.href = url;
downloadLink.download = 'demo.mp4'; // Specify the desired filename
// Programmatically trigger a click event on the anchor to initiate the download
downloadLink.click();
}
// Listen for messages from content / popup
chrome.runtime.onMessage.addListener(
function(request, sender, sendResponse) {
console.log(request)
if(request.type =="tabRecord"){
recordTab(request.streamId,request.tabId);
sendResponse("startRecord");
}
if(request.type == "stopRecording"){
mediaRecorder.stop();
sendResponse("stopRecord");
}
}
);
// record the tab only
async function recordTab(streamId,tabId){
config = {
"video": {
"mandatory": {
"chromeMediaSourceId": streamId,
"chromeMediaSource": "tab"
}
},
"audio": {
"mandatory": {
"chromeMediaSourceId": streamId,
"chromeMediaSource": "tab"
}
}
}
navigator.mediaDevices.getUserMedia(config).then(function (desktop) {
var stream = new MediaStream();
if(desktop){
video = desktop.getVideoTracks()[0];
stream.addTrack(video);
}
mediaRecorder = new MediaRecorder(stream, {
mimeType: 'video/webm;codecs=h264',
});
recordedChunks = [];
mediaRecorder.onstop=function(){
var tracks = {};
tracks.a = stream ? stream.getTracks() : [];
tracks.b = desktop ? desktop.getTracks() : [];
tracks.c = [];
tracks.total = [...tracks.a, ...tracks.b,...tracks.c];
/* */
for (var i = 0; i < tracks.total.length; i++) {
if (tracks.total[i]) {
tracks.total[i].stop();
}
}
stopRecord(recordedChunks)
}
mediaRecorder.ondataavailable = e => {
if (e.data.size > 0) {
recordedChunks.push(e.data);
}
};
stream.onended = function(){
mediaRecorder.stop()
}
mediaRecorder.start()
})
}
- Load the extension in Chrome:
- Open Chrome browser
- Go to
chrome://extensions
- Enable “Developer mode”
- Click on “Load unpacked”
- Select the folder containing your extension files
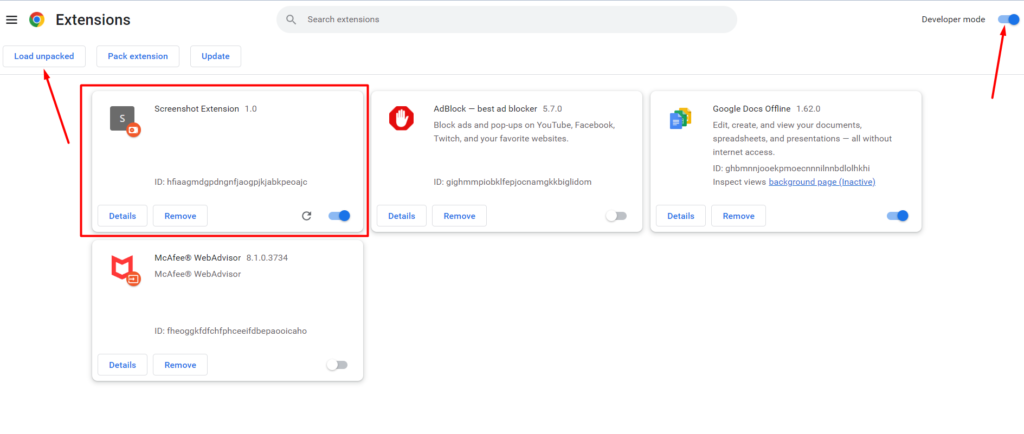
Let’s break down the code step by step:
- Variable Declarations:
var mediaRecorder;
var recordedChunks = [];
var seconds = 0;
Here, you declare three variables: mediaRecorder
, recordedChunks
, and seconds
. These will be used to manage the recording process and track the recorded data.
stopRecord
Function:
function stopRecord(recordedChunks) {
if (mediaRecorder.state == 'recording') {
mediaRecorder.stop();
}
// ... (code for creating a download link for the recorded video)
}
This function is responsible for stopping the recording process. It checks if the mediaRecorder
is in the ‘recording’ state and stops it. Then, it creates a Blob from the recorded chunks and generates a download link for the video.
- Message Listener:
chrome.runtime.onMessage.addListener(function (request, sender, sendResponse) {
// ... (code for handling messages from the extension's popup or content script)
});
This code sets up a message listener using the chrome.runtime.onMessage.addListener
method. It listens for messages from the extension’s popup or content script.
recordTab
Function:
async function recordTab(streamId, tabId) {
// ... (code for configuring and starting recording of the current tab)
}
This function is responsible for recording the current tab. It takes a streamId
and tabId
as parameters and uses the navigator.mediaDevices.getUserMedia
method to access the tab’s audio and video streams. It sets up a MediaRecorder
to capture the video and audio data from the tab.
- Initialization of
mediaRecorder
and Event Handlers:
Inside therecordTab
function, there is code for initializing themediaRecorder
object and setting up event handlers to capture data and handle the stop event. - Cleanup and Stopping the Recording:
When the recording is stopped, whether manually or when the tab’s stream ends, themediaRecorder.onstop
event handler is triggered. It stops all tracks associated with the recording, including video and audio tracks, and then calls thestopRecord
function to generate the download link for the recorded video.
The source code for this project is available on Github.